遇到中间表怎么实现搜索中间表,追加属性
框架中如 group_name_arr
开启搜索功能后,如何才能实现搜索?请以这个为例给一个实际的解决方案:
language
{ label: t('auth.admin.grouping'), prop: 'group_name_arr', align: 'center', operator: 'LIKE', render: 'tags' },
language
public function getGroupNameArrAttr($value, $row): array
{
$groupAccess = Db::name('admin_group_access')
->where('uid', $row['id'])
->column('group_id');
return AdminGroup::whereIn('id', $groupAccess)->column('name');
}
2个回答默认排序 投票数排序
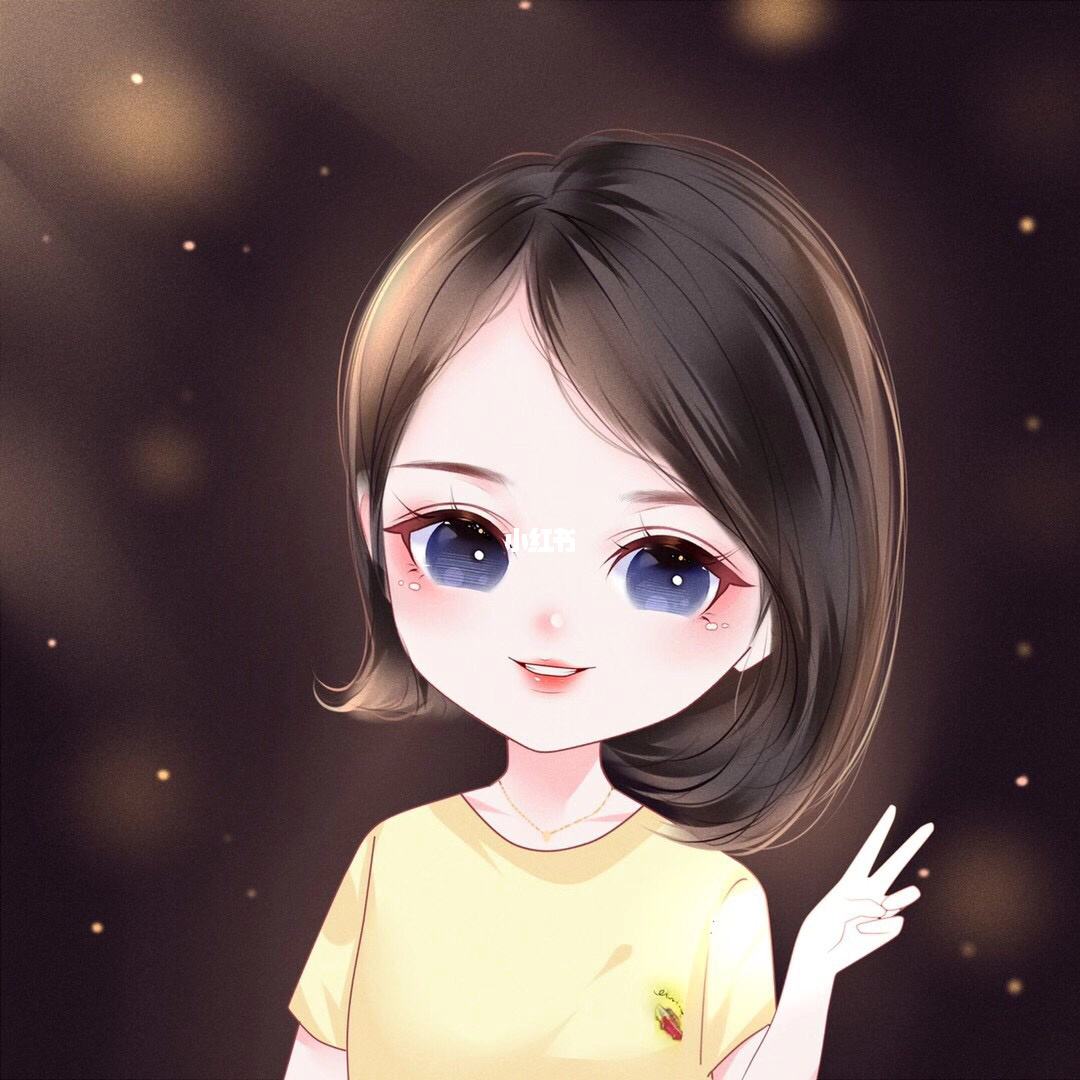
siccd
这家伙很懒,什么也没写~
1天前
给一个完整的示例供大家参考
创建 AdminGroupAccess.php 空模型
language
<?php
namespace app\admin\model;
use think\Model;
/**
* AdminGroupAccess模型
*/
class AdminGroupAccess extends Model
{
protected $autoWriteTimestamp = true;
}
创建 AdminGroupAccess.php 空的控制器
language
<?php
namespace app\admin\controller\auth;
use app\common\controller\Backend;
/**
* 管理分组映射表
*/
class AdminGroupAccess extends Backend
{
/**
* AdminGroupAccess模型对象
* @var object
* @phpstan-var \app\admin\model\AdminGroupAccess
*/
protected object $model;
protected array|string $preExcludeFields = ['id'];
protected string|array $quickSearchField = ['id'];
public function initialize(): void
{
parent::initialize();
$this->model = new \app\admin\model\AdminGroupAccess();
}
/**
* 若需重写查看、编辑、删除等方法,请复制 @see \app\admin\library\traits\Backend 中对应的方法至此进行重写
*/
}
Admin.php 模型
language
<?php
namespace app\admin\model;
use ba\Random;
use think\Model;
use think\facade\Db;
/**
* Admin模型
* @property int $id 管理员ID
* @property string $username 管理员用户名
* @property string $nickname 管理员昵称
* @property string $email 管理员邮箱
* @property string $mobile 管理员手机号
* @property string $last_login_ip 上次登录IP
* @property string $last_login_time 上次登录时间
* @property int $login_failure 登录失败次数
*/
class Admin extends Model
{
/**
* @var string 自动写入时间戳
*/
protected $autoWriteTimestamp = true;
/**
* 追加属性
*/
protected $append = [
'admin_group',
'admin_group_access',
];
public function getAvatarAttr($value): string
{
return full_url($value, false, config('buildadmin.default_avatar'));
}
public function setAvatarAttr($value): string
{
return $value == full_url('', false, config('buildadmin.default_avatar')) ? '' : $value;
}
public function getLastLoginTimeAttr($value): string
{
return $value ? date('Y-m-d H:i:s', $value) : '';
}
/**
* 重置用户密码
* @param int|string $uid 管理员ID
* @param string $newPassword 新密码
* @return int|Admin
*/
public function resetPassword(int|string $uid, string $newPassword): int|Admin
{
$salt = Random::build('alnum', 16);
$passwd = encrypt_password($newPassword, $salt);
return $this->where(['id' => $uid])->update(['password' => $passwd, 'salt' => $salt]);
}
public function adminGroup()
{
return $this->belongsToMany(
\app\admin\model\AdminGroup::class, // 目标模型:用户组
'admin_group_access', // 中间表名
'group_id', // 中间表关联目标模型(AdminGroup)的外键
'uid', // 中间表关联当前模型(Admin)的外键
)->hidden(['pivot']); // 添加此行隐藏pivot属性
}
public function adminGroupAccess(): \think\model\relation\HasOne
{
return $this->hasOne(AdminGroupAccess::class, 'uid', 'id');
}
}
admin.php控制器
language
<?php
namespace app\admin\controller\auth;
use ba\Random;
use Throwable;
use think\facade\Db;
use app\common\controller\Backend;
use app\admin\model\Admin as AdminModel;
class Admin extends Backend
{
/**
* 模型
* @var object
* @phpstan-var AdminModel
*/
protected object $model;
protected array $noNeedPermission = ['index'];
protected array $withJoinTable = ['admin_group_access'];
protected array|string $preExcludeFields = ['create_time', 'update_time', 'password', 'salt', 'login_failure', 'last_login_time', 'last_login_ip'];
protected array|string $quickSearchField = ['username', 'nickname'];
/**
* 开启数据限制
*/
protected string|int|bool $dataLimit = 'allAuthAndOthers';
protected string $dataLimitField = 'id';
public function initialize(): void
{
parent::initialize();
$this->model = new AdminModel();
}
/**
* 查看
* @throws Throwable
*/
public function index(): void
{
if ($this->request->param('select')) {
$this->select();
}
list($where, $alias, $limit, $order) = $this->queryBuilder();
$res = $this->model
->withoutField('login_failure,password,salt')
->withJoin($this->withJoinTable, $this->withJoinType)
->alias($alias)
->where($where)
->order($order)
->group('admin.id')
->paginate($limit);
$res->hidden(['admin_group_access'])->visible([
'adminGroup' => ['id','name'],
]);
$this->success('', [
'list' => $res->items(),
'total' => $res->total(),
'remark' => get_route_remark(),
]);
}
/**
* 添加
* @throws Throwable
*/
public function add(): void
{
if ($this->request->isPost()) {
$data = $this->request->post();
if (!$data) {
$this->error(__('Parameter %s can not be empty', ['']));
}
/**
* 由于有密码字段-对方法进行重写
* 数据验证
*/
if ($this->modelValidate) {
try {
$validate = str_replace("\\model\\", "\\validate\\", get_class($this->model));
$validate = new $validate();
$validate->scene('add')->check($data);
} catch (Throwable $e) {
$this->error($e->getMessage());
}
}
$salt = Random::build('alnum', 16);
$passwd = encrypt_password($data['password'], $salt);
$data = $this->excludeFields($data);
$result = false;
if ($data['group_arr']) $this->checkGroupAuth($data['group_arr']);
$this->model->startTrans();
try {
$data['salt'] = $salt;
$data['password'] = $passwd;
$result = $this->model->save($data);
if ($data['group_arr']) {
$groupAccess = [];
foreach ($data['group_arr'] as $datum) {
$groupAccess[] = [
'uid' => $this->model->id,
'group_id' => $datum,
];
}
Db::name('admin_group_access')->insertAll($groupAccess);
}
$this->model->commit();
} catch (Throwable $e) {
$this->model->rollback();
$this->error($e->getMessage());
}
if ($result !== false) {
$this->success(__('Added successfully'));
} else {
$this->error(__('No rows were added'));
}
}
$this->error(__('Parameter error'));
}
/**
* 编辑
* @throws Throwable
*/
public function edit(): void
{
$pk = $this->model->getPk();
$id = $this->request->param($pk);
$row = $this->model->find($id);
if (!$row) {
$this->error(__('Record not found'));
}
$dataLimitAdminIds = $this->getDataLimitAdminIds();
if ($dataLimitAdminIds && !in_array($row[$this->dataLimitField], $dataLimitAdminIds)) {
$this->error(__('You have no permission'));
}
if ($this->request->isPost()) {
$data = $this->request->post();
if (!$data) {
$this->error(__('Parameter %s can not be empty', ['']));
}
/**
* 由于有密码字段-对方法进行重写
* 数据验证
*/
if ($this->modelValidate) {
try {
$validate = str_replace("\\model\\", "\\validate\\", get_class($this->model));
$validate = new $validate();
$validate->scene('edit')->check($data);
} catch (Throwable $e) {
$this->error($e->getMessage());
}
}
if ($this->auth->id == $data['id'] && $data['status'] == '0') {
$this->error(__('Please use another administrator account to disable the current account!'));
}
if (isset($data['password']) && $data['password']) {
$this->model->resetPassword($data['id'], $data['password']);
}
$groupAccess = [];
if ($data['group_arr']) {
$checkGroups = [];
foreach ($data['group_arr'] as $datum) {
if (!in_array($datum, $row->group_arr)) {
$checkGroups[] = $datum;
}
$groupAccess[] = [
'uid' => $id,
'group_id' => $datum,
];
}
$this->checkGroupAuth($checkGroups);
}
Db::name('admin_group_access')
->where('uid', $id)
->delete();
$data = $this->excludeFields($data);
$result = false;
$this->model->startTrans();
try {
$result = $row->save($data);
if ($groupAccess) Db::name('admin_group_access')->insertAll($groupAccess);
$this->model->commit();
} catch (Throwable $e) {
$this->model->rollback();
$this->error($e->getMessage());
}
if ($result !== false) {
$this->success(__('Update successful'));
} else {
$this->error(__('No rows updated'));
}
}
unset($row['salt'], $row['login_failure']);
$row['password'] = '';
$this->success('', [
'row' => $row
]);
}
/**
* 删除
* @throws Throwable
*/
public function del(): void
{
$where = [];
$dataLimitAdminIds = $this->getDataLimitAdminIds();
if ($dataLimitAdminIds) {
$where[] = [$this->dataLimitField, 'in', $dataLimitAdminIds];
}
$ids = $this->request->param('ids/a', []);
$where[] = [$this->model->getPk(), 'in', $ids];
$data = $this->model->where($where)->select();
$count = 0;
$this->model->startTrans();
try {
foreach ($data as $v) {
if ($v->id != $this->auth->id) {
$count += $v->delete();
Db::name('admin_group_access')
->where('uid', $v['id'])
->delete();
}
}
$this->model->commit();
} catch (Throwable $e) {
$this->model->rollback();
$this->error($e->getMessage());
}
if ($count) {
$this->success(__('Deleted successfully'));
} else {
$this->error(__('No rows were deleted'));
}
}
/**
* 检查分组权限
* @throws Throwable
*/
private function checkGroupAuth(array $groups): void
{
if ($this->auth->isSuperAdmin()) {
return;
}
$authGroups = $this->auth->getAllAuthGroups('allAuthAndOthers');
foreach ($groups as $group) {
if (!in_array($group, $authGroups)) {
$this->error(__('You have no permission to add an administrator to this group!'));
}
}
}
}
前端index.vue
language
<template>
<div class="default-main ba-table-box">
<el-alert class="ba-table-alert" v-if="baTable.table.remark" :title="baTable.table.remark" type="info" show-icon />
<!-- 表格顶部菜单 -->
<TableHeader
:buttons="['refresh', 'add', 'edit', 'delete', 'comSearch', 'quickSearch', 'columnDisplay']"
:quick-search-placeholder="t('Quick search placeholder', { fields: t('auth.admin.username') + '/' + t('auth.admin.nickname') })"
/>
<!-- 表格 -->
<!-- 要使用`el-table`组件原有的属性,直接加在Table标签上即可 -->
<Table>
<template #admin_group>
<el-table-column prop="admin_group" align="center" :label="t('auth.admin.grouping')">
<template #default="scope">
<el-tag v-for="(item, index) in scope.row.admin_group" :key="index">
{{ item.name }}
</el-tag>
</template>
</el-table-column>
</template>
</Table>
<!-- 表单 -->
<PopupForm />
</div>
</template>
cleanXss
import { provide } from 'vue'
import baTableClass from '/@/utils/baTable'
import PopupForm from './popupForm.vue'
import Table from '/@/components/table/index.vue'
import TableHeader from '/@/components/table/header/index.vue'
import { defaultOptButtons } from '/@/components/table'
import { baTableApi } from '/@/api/common'
import { useAdminInfo } from '/@/stores/adminInfo'
import { useI18n } from 'vue-i18n'
defineOptions({
name: 'auth/admin',
})
const { t } = useI18n()
const adminInfo = useAdminInfo()
const optButtons = defaultOptButtons(['edit', 'delete'])
optButtons[1].display = (row) => {
return row.id != adminInfo.id
}
const baTable = new baTableClass(
new baTableApi('/admin/auth.Admin/'),
{
column: [
{ type: 'selection', align: 'center', operator: false },
{ label: t('Id'), prop: 'id', align: 'center', operator: '=', operatorPlaceholder: t('Id'), width: 70 },
{ label: t('auth.admin.username'), prop: 'username', align: 'center', operator: 'LIKE', operatorPlaceholder: t('Fuzzy query') },
{ label: t('auth.admin.nickname'), prop: 'nickname', align: 'center', operator: 'LIKE', operatorPlaceholder: t('Fuzzy query') },
{
label: t('auth.admin.grouping'),
render: 'slot',
slotName: 'admin_group',
operator: false,
},
{
label: t('auth.admin.grouping'),
prop: 'admin_group_access.group_id',
align: 'center',
operator: 'FIND_IN_SET',
show: false,
comSearchRender: 'remoteSelect',
remote: {
pk: 'admin_group.id',
field: 'name',
remoteUrl: '/admin/auth.Group/index',
},
enableColumnDisplayControl: false,
},
{ label: t('auth.admin.head portrait'), prop: 'avatar', align: 'center', render: 'image', operator: false },
{ label: t('auth.admin.mailbox'), prop: 'email', align: 'center', operator: 'LIKE', operatorPlaceholder: t('Fuzzy query') },
{ label: t('auth.admin.mobile'), prop: 'mobile', align: 'center', operator: 'LIKE', operatorPlaceholder: t('Fuzzy query') },
{
label: t('auth.admin.Last login'),
prop: 'last_login_time',
align: 'center',
render: 'datetime',
sortable: 'custom',
operator: 'RANGE',
width: 160,
},
{ label: t('Create time'), prop: 'create_time', align: 'center', render: 'datetime', sortable: 'custom', operator: 'RANGE', width: 160 },
{
label: t('State'),
prop: 'status',
align: 'center',
render: 'tag',
custom: { '0': 'danger', '1': 'success' },
replaceValue: { '0': t('Disable'), '1': t('Enable') },
},
{
label: t('Operate'),
align: 'center',
width: '100',
render: 'buttons',
buttons: optButtons,
operator: false,
},
],
dblClickNotEditColumn: [undefined, 'status'],
},
{
defaultItems: {
status: '1',
},
}
)
provide('baTable', baTable)
baTable.mount()
baTable.getIndex()
cleanXss
<style scoped lang="scss"></style>
以上是完整的代码。
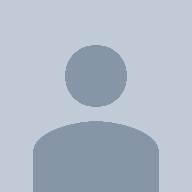
xiaoyangyang
这家伙很懒,什么也没写~
1天前
文档里面有
请先登录
后台可视化 CRUD,生成一个远程下拉(多选),自己看